Unlocking the Power of Retrieval-Augmented Generation: A Step-by-Step Guide
Explore the potential of Retrieval-Augmented Generation (RAG) using large language models (LLMs) with larger context windows and learn how to integrate FAISS for efficient document retrieval.
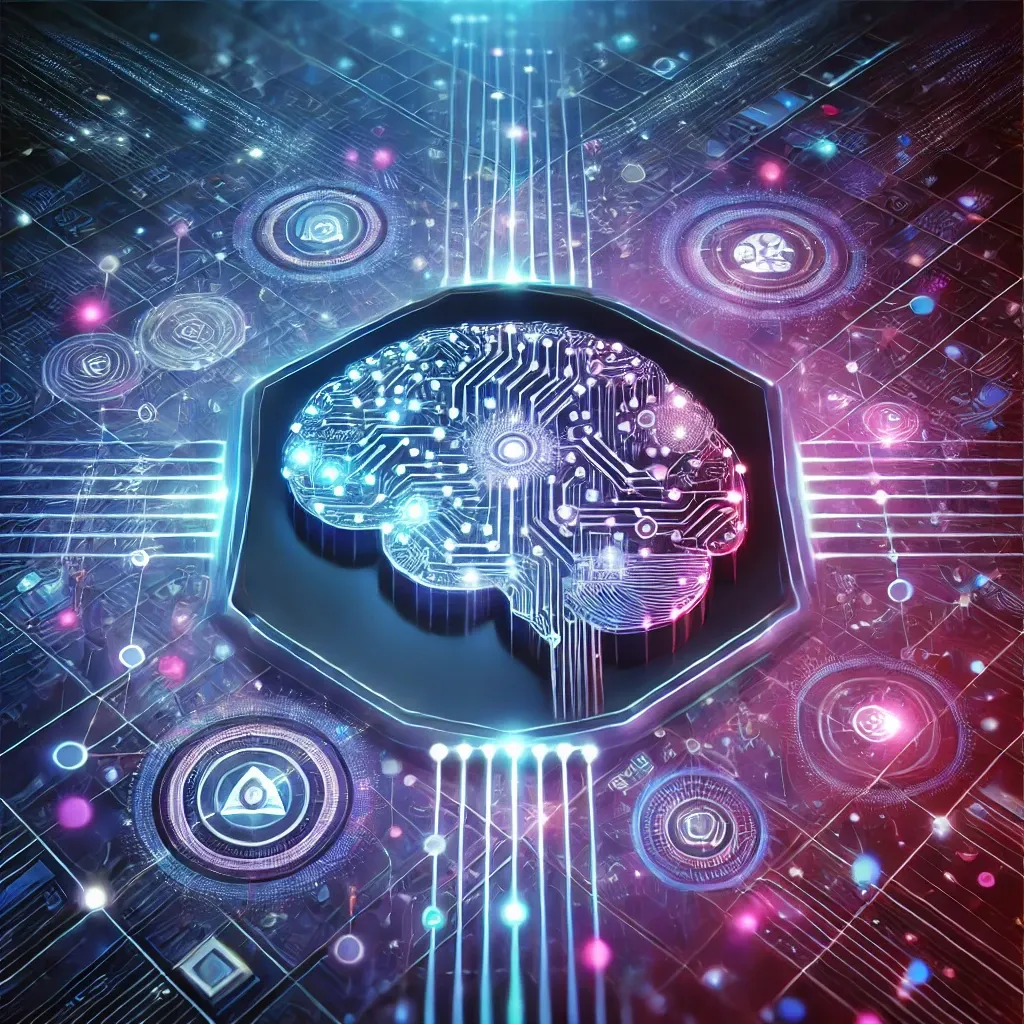
Introduction
The rapid evolution of large language models (LLMs) like GPT-4 and BERT has transformed the field of natural language processing (NLP). These models are capable of generating highly sophisticated and contextually accurate text, but they have inherent limitations, particularly when recalling specific or vast amounts of information. To overcome these limitations, a technique known as Retrieval-Augmented Generation (RAG) has emerged. RAG combines the generative capabilities of LLMs with the ability to retrieve relevant documents from external sources, leading to more informed and accurate text generation.
In this comprehensive guide, we'll explore how RAG works, the importance of using tokenizers with larger context windows in LLMs, and how to integrate FAISS (Facebook AI Similarity Search) for efficient document retrieval. We'll provide step-by-step Python examples to illustrate how you can implement these concepts in your own projects.
Understanding Retrieval-Augmented Generation (RAG)
What is Retrieval-Augmented Generation?
Retrieval-Augmented Generation (RAG) is an advanced NLP technique that enhances the performance of LLMs by enabling them to access and retrieve relevant documents from external knowledge bases before generating text. Instead of relying solely on the information encoded during training, RAG allows models to augment their responses with up-to-date, contextually relevant information retrieved from external sources.
The Role of Retrieval in LLMs
While LLMs like GPT-4 are highly effective in generating coherent and contextually appropriate text, they are limited by the data they were trained on and the fixed size of their context windows (the number of tokens they can process simultaneously). RAG addresses these limitations by incorporating a retrieval mechanism that queries external databases or knowledge repositories for relevant information, which is then used to inform and enhance the model's output.
Leveraging Tokenizers with Larger Context Windows
The Importance of Tokenization
Tokenization is the process of converting text into smaller units, or tokens, that an LLM can process. The size of the context window—the maximum number of tokens the model can handle—directly impacts the model's ability to generate accurate and contextually rich responses. Larger context windows allow models to process more information at once, leading to better integration of retrieved content and more coherent outputs.
Why Larger Context Windows Matter in RAG
In the context of RAG, larger context windows are crucial. They allow the model to seamlessly integrate more of the retrieved documents with the original input prompt, leading to higher quality text generation. This is particularly important when dealing with complex queries that require the model to consider multiple pieces of evidence or detailed explanations.
Implementing Tokenizers with Larger Context Windows
To implement tokenizers with larger context windows in Python, we can use the Hugging Face Transformers library. This library provides pre-trained tokenizers and models that can be adapted to support larger context windows, facilitating the RAG process.
Step 1: Install the Required Libraries
!pip install transformers
Step 2: Load the Tokenizer and Model
from transformers import AutoTokenizer, AutoModelForSeq2SeqLM
# Load a model and tokenizer that supports a larger context window
tokenizer = AutoTokenizer.from_pretrained('gpt-3.5-turbo')
model = AutoModelForSeq2SeqLM.from_pretrained('gpt-3.5-turbo')
Step 3: Adjust the Tokenizer for a Larger Context Window
# Increase the maximum length for tokenization
tokenizer.model_max_length = 4096 # Setting the context window to 4096 tokens
Step 4: Tokenize and Process Input with a Larger Context
# Example input text and retrieval results
input_text = "Explain the significance of quantum computing."
retrieved_docs = ["Quantum computing leverages the principles of quantum mechanics...",
"The superposition and entanglement properties in quantum computing..."]
# Combine the input with retrieved documents
combined_input = input_text + " ".join(retrieved_docs)
# Tokenize the combined input
tokens = tokenizer(combined_input, return_tensors='pt', truncation=True, padding='max_length')
# Generate the response using the model
output = model.generate(**tokens, max_length=500)
response = tokenizer.decode(output[0], skip_special_tokens=True)
print(response)
This example demonstrates how to combine the input prompt with retrieved documents and tokenize the entire text within the model's extended context window. This process allows the LLM to generate a response that is informed by the additional context provided by the retrieved documents.
Integrating Document Retrieval with FAISS
Overview of FAISS
FAISS (Facebook AI Similarity Search) is a powerful library for efficient similarity search and clustering of dense vectors. It is commonly used in NLP to quickly retrieve documents that are most similar to a given query, based on their dense embeddings. Integrating FAISS with RAG allows for fast and accurate retrieval of relevant documents, which can then be used to augment the text generation process.
Using the Same Tokenizer for LLMs and FAISS
Using the same tokenizer for both the LLM and the document retrieval system, including FAISS, ensures consistency in how text is processed across the entire pipeline. This consistency is crucial for the effective implementation of RAG, as it ensures that the documents and queries are encoded in a compatible manner.
Step 1: Install Necessary Libraries
!pip install transformers faiss-cpu
Step 2: Load the Tokenizer and Model
from transformers import AutoTokenizer, AutoModel
import faiss
import numpy as np
# Load a pre-trained tokenizer and model (e.g., BERT)
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
Step 3: Tokenize and Encode Documents
To use FAISS, you need to convert your documents into dense vectors. First, tokenize the documents using the same tokenizer, and then pass them through the model to obtain embeddings.
# Example documents
documents = [
"Quantum computing leverages quantum mechanics...",
"Classical computers use binary encoding...",
"Quantum computers utilize qubits for processing...",
# More documents
]
# Tokenize the documents
tokenized_docs = tokenizer(documents, padding=True, truncation=True, return_tensors='pt')
# Encode documents into dense vectors using the model
with torch.no_grad():
model_output = model(**tokenized_docs)
embeddings = model_output.last_hidden_state[:, 0, :].numpy() # Extract [CLS] token embeddings
Step 4: Create a FAISS Index and Add Embeddings
Once you have the embeddings, you can create a FAISS index and add these vectors for efficient retrieval.
# Create a FAISS index
d = embeddings.shape[1] # Dimension of the embeddings
index = faiss.IndexFlatL2(d) # L2 distance (Euclidean)
# Add the document embeddings to the FAISS index
index.add(embeddings)
# Now, you can perform searches on the index
Step 5: Retrieve Documents Based on a Query
To retrieve documents, tokenize and encode the query in the same way as the documents, and search the FAISS index for the most similar documents.
# Example query
query = "How does quantum computing work?"
# Tokenize and encode the query
tokenized_query = tokenizer(query, return_tensors='pt')
with torch.no_grad():
query_embedding = model(**tokenized_query).last_hidden_state[:, 0, :].numpy()
# Search the FAISS index for the most similar documents
D, I = index.search(query_embedding, k=3) # k is the number of top results to retrieve
# Retrieve the most similar documents
retrieved_docs = [documents[i] for i in I[0]]
print(retrieved_docs)
In this example, the retrieve_docs
function returns the top-k most relevant documents based on the input query. These documents are then combined with the query and passed to the language model for generating the final response.
Benefits of Using the Same Tokenizer
- Consistency: Ensures that both the LLM and the retrieval system process text in the same way, reducing potential mismatches.
- Efficiency: Streamlines the pipeline by using a unified tokenization process, which is especially important when scaling RAG to handle large datasets.
- Enhanced Retrieval: Improves the quality of retrieved documents and the subsequent text generation by maintaining uniformity in text representation.
Challenges and Considerations
Computational Resources
Implementing RAG with large context windows and FAISS can be resource-intensive, requiring significant memory and processing power, especially when dealing with large datasets or complex queries.
Balancing Relevance and Context
While larger context windows allow for more comprehensive processing, it's essential to balance the relevance of retrieved documents with the overall context. Including too much information can dilute the quality of the generated text, leading to off-topic or irrelevant responses.
Index Management in FAISS
As you add more documents to your FAISS index, it can become large and complex, requiring careful management of memory and storage to maintain retrieval efficiency.
Future Directions in RAG and LLMs
Dynamic Context Windows
Future developments may include dynamic context windows, where the model adjusts the size of its context window based on the complexity of the task or query, optimizing resource use and improving performance.
Advanced Retrieval Mechanisms
Integrating more sophisticated retrieval mechanisms, such as dense retrieval, knowledge graphs, or hybrid models that combine multiple strategies, can further enhance the relevance and accuracy of retrieved documents.
FAQs
How does Retrieval-Augmented Generation improve language models?
Retrieval-Augmented Generation improves language models by enabling them to access and integrate external knowledge during text generation, resulting in more accurate, contextually relevant, and up-to-date responses.
What role do tokenizers play in RAG?
Tokenizers break down text into smaller units (tokens) that the model processes. In RAG, tokenizers with larger context windows allow models to consider more information simultaneously, improving the quality of generated content.
Why are larger context windows important for RAG?
Larger context windows allow models to process more tokens at once, which is crucial for integrating retrieved documents with the original input prompt. This leads to better contextual understanding and more accurate text generation.
What are the challenges of using RAG with large LLMs?
Challenges include the increased computational resources required for larger context windows and the need to balance the relevance of retrieved documents with the context to avoid off-topic or irrelevant generation.
How can we improve the retrieval process in RAG?
Improvements can be made by using advanced retrieval techniques like dense retrieval, knowledge graphs, or hybrid models that combine multiple retrieval strategies for more accurate and relevant document retrieval.
Can RAG be applied to other tasks beyond text generation?
Yes, RAG can be applied to various tasks, including question answering, summarization, and any task where the integration of external knowledge can enhance the performance of language models.
Conclusion
Retrieval-Augmented Generation represents a significant advancement in the field of NLP, allowing large language models to access and integrate external knowledge during the text generation process. By leveraging tokenizers with larger context windows and integrating FAISS for efficient document retrieval, we can dramatically improve the accuracy and relevance of generated content. As both LLMs and retrieval systems continue to evolve, the potential applications of RAG will expand, offering even more sophisticated and contextually aware text generation solutions.
This blog post provides a detailed exploration of how to implement and leverage Retrieval-Augmented Generation (RAG) using large language models, tokenizers with larger context windows, and FAISS for efficient document retrieval. By following the outlined steps and considerations, you can create powerful NLP systems that combine the best of both generative and retrieval-based approaches.