Overview of Clean Code
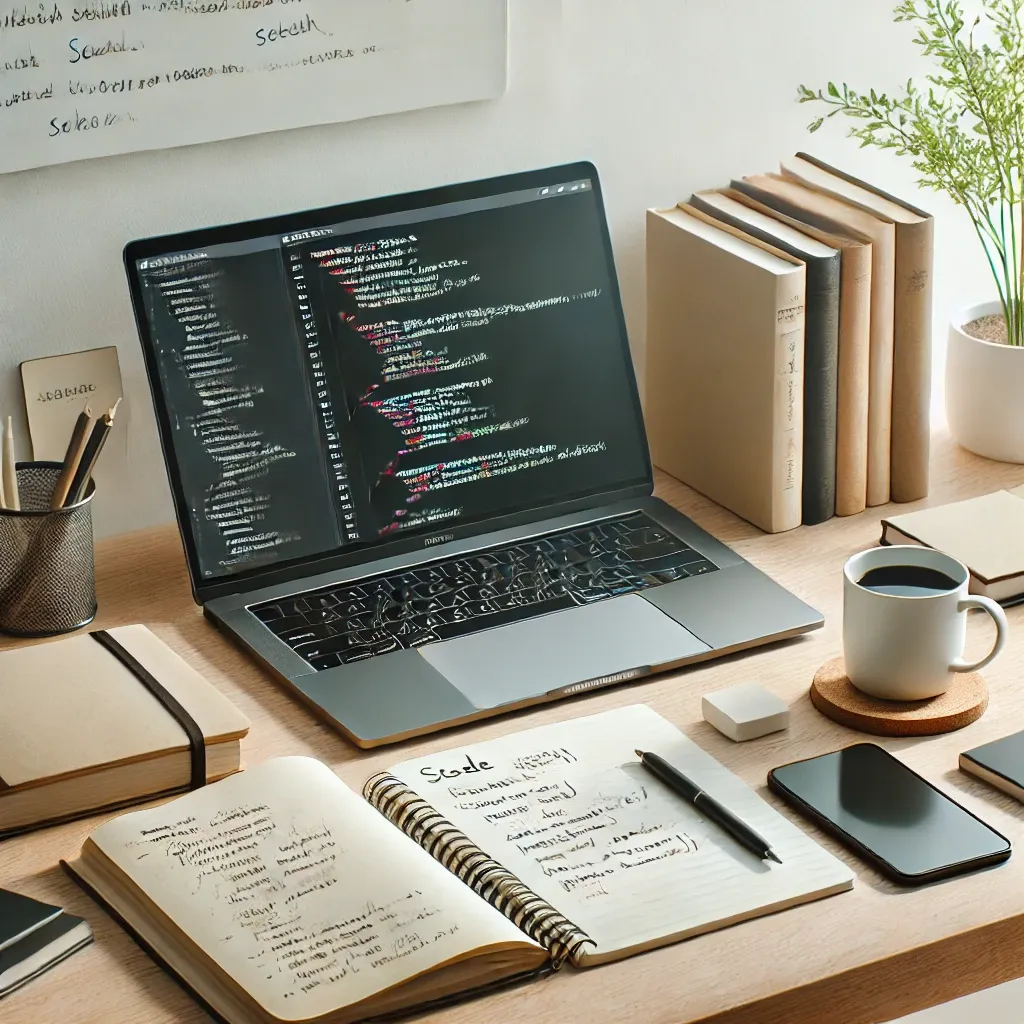
Introduction
Clean code is a philosophy and a set of principles for writing code that is easy to read, maintain, and extend. It was popularized by Robert C. Martin (Uncle Bob) in his book “Clean Code: A Handbook of Agile Software Craftsmanship.”
Why Clean Code?
- Readability: Clean code is easy to read and understand.
- Maintainability: It is easier to maintain and update.
- Scalability: Well-structured code can scale more effectively.
- Bug Reduction: Cleaner code tends to have fewer bugs.
Principles of Clean Code
- Meaningful Names: Use descriptive and meaningful names for variables, functions, and classes.
- Single Responsibility Principle (SRP): Each class or function should have one and only one responsibility.
- DRY (Don’t Repeat Yourself): Avoid duplication of code.
- KISS (Keep It Simple, Stupid): Keep the code simple and straightforward.
- YAGNI (You Aren’t Gonna Need It): Don’t add functionality until it is necessary.
Example
# Bad Code
def d1(lst):
a = []
for i in lst:
if i % 2 == 0:
a.append(i)
return a
# Clean Code
def filter_even_numbers(numbers):
even_numbers = [number for number in numbers if number % 2 == 0]
return even_numbers
Conclusion
Clean code is essential for developing robust and maintainable software. By following the principles of clean code, developers can create systems that are easier to understand, modify, and scale.