Implementing a Text and Image Multimodal Large Language Model: An End-to-End Guide
Learn how to implement a multimodal Large Language Model that integrates text and image inputs. This blog walks through the end-to-end process, from data preparation to model deployment, providing a practical guide for developers.
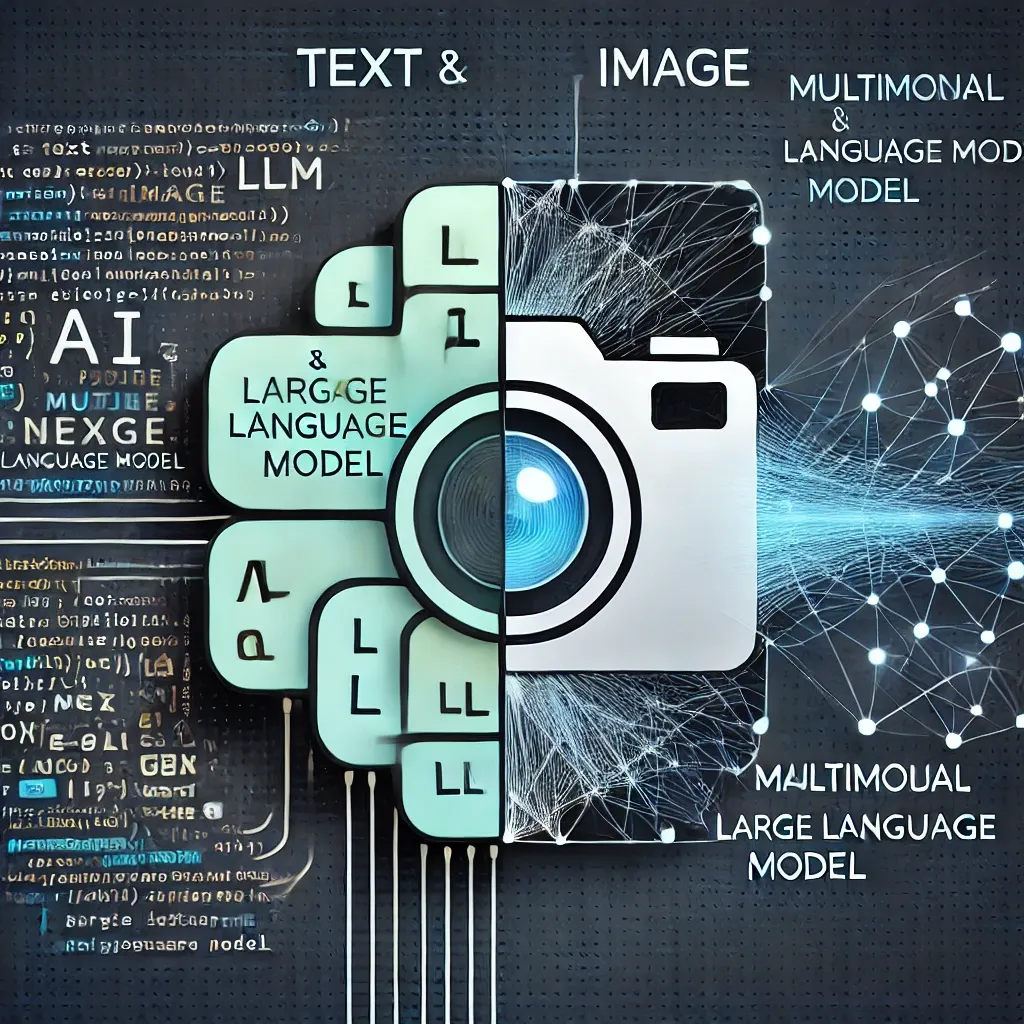
Introduction
Multimodal Large Language Models (LLMs) that can process both text and images have become a powerful tool in the AI community. These models have the ability to understand and generate outputs that integrate information from both text and visual data, making them invaluable for applications like image captioning, visual question answering, and more. In this blog post, we will walk through an end-to-end example of how to implement a text and image multimodal LLM, covering everything from data preparation to model deployment.
Step 1: Defining the Use Case
Before diving into implementation, it's important to define the use case for your multimodal LLM. Let's assume we want to build a model that can generate descriptive captions for images—a common application of multimodal models.
Our goal is to create a model that, given an image and an optional text prompt, can generate a caption that accurately describes the content of the image. This use case will guide our choices throughout the implementation process.
Step 2: Data Collection and Preparation
To train a multimodal LLM, you'll need a dataset that includes paired text and image data. A popular choice for this task is the COCO dataset, which contains a large number of images annotated with descriptive captions.
2.1 Downloading the Dataset
You can start by downloading the COCO dataset. The dataset typically includes the following components:
- Images: A collection of images in various categories.
- Captions: Textual descriptions corresponding to each image.
2.2 Preprocessing the Data
Preprocessing is crucial to ensure that the data is in a suitable format for model training. Here's how you can preprocess the text and images:
- Text Preprocessing: Tokenize the captions using a tokenizer such as Byte-Pair Encoding (BPE) or WordPiece. Ensure that the captions are padded to a uniform length, which simplifies batch processing.
- Image Preprocessing: Resize the images to a standard size (e.g., 224x224 pixels) and normalize pixel values to be within a certain range (typically [0, 1] or [-1, 1]). You may also want to apply data augmentation techniques like random cropping, flipping, and rotation to increase the diversity of your training data.
Here’s a snippet in Python for basic preprocessing:
from PIL import Image
import torchvision.transforms as transforms
from transformers import AutoTokenizer
# Image preprocessing
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]),
])
def preprocess_image(image_path):
image = Image.open(image_path).convert('RGB')
return transform(image)
# Text preprocessing
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
def preprocess_caption(caption):
return tokenizer(caption, padding='max_length', truncation=True, return_tensors="pt")
Step 3: Model Architecture
Now that our data is ready, it’s time to design the model architecture. The model needs to process both text and images, extract meaningful features, and then integrate these features to generate a caption.
3.1 Text Encoder
For the text input, we can use a pre-trained transformer model like BERT. This model will take a caption (or prompt) as input and output a sequence of embeddings that represent the semantic content of the text.
from transformers import BertModel
text_encoder = BertModel.from_pretrained('bert-base-uncased')
def encode_text(text_input):
text_output = text_encoder(**text_input)
return text_output.last_hidden_state
3.2 Image Encoder
For the image input, a pre-trained convolutional neural network (CNN) such as ResNet or a Vision Transformer (ViT) can be used to extract visual features. These features are typically in the form of a high-dimensional vector.
import torch.nn as nn
import torchvision.models as models
class ImageEncoder(nn.Module):
def __init__(self):
super(ImageEncoder, self).__init__()
self.resnet = models.resnet50(pretrained=True)
self.resnet.fc = nn.Identity() # Remove the final classification layer
def forward(self, images):
return self.resnet(images)
image_encoder = ImageEncoder()
3.3 Multimodal Fusion
The core challenge in a multimodal model is combining the text and image features into a unified representation. One approach is to concatenate the features and pass them through a series of fully connected layers.
class MultimodalModel(nn.Module):
def __init__(self, text_encoder, image_encoder):
super(MultimodalModel, self).__init__()
self.text_encoder = text_encoder
self.image_encoder = image_encoder
self.fc = nn.Sequential(
nn.Linear(2048 + 768, 1024),
nn.ReLU(),
nn.Linear(1024, 512),
nn.ReLU(),
nn.Linear(512, 256),
nn.ReLU(),
nn.Linear(256, vocab_size) # vocab_size = size of the tokenizer vocabulary
)
def forward(self, text_input, images):
text_features = self.text_encoder(text_input)
image_features = self.image_encoder(images)
combined_features = torch.cat((text_features[:, 0, :], image_features), dim=1)
output = self.fc(combined_features)
return output
Step 4: Training the Model
With the architecture in place, the next step is to train the model. This involves defining a loss function, setting up an optimizer, and running the training loop.
4.1 Loss Function
For image captioning, the loss function is typically the cross-entropy loss between the predicted tokens and the ground truth tokens in the caption.
criterion = nn.CrossEntropyLoss()
4.2 Optimizer
The optimizer updates the model weights based on the gradients computed during backpropagation. A common choice is Adam.
optimizer = torch.optim.Adam(model.parameters(), lr=1e-4)
4.3 Training Loop
The training loop iteratively feeds batches of data into the model, computes the loss, and updates the model weights.
num_epochs = 10
for epoch in range(num_epochs):
for batch in dataloader:
text_input, images, captions = batch
optimizer.zero_grad()
output = model(text_input, images)
loss = criterion(output, captions)
loss.backward()
optimizer.step()
print(f'Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}')
Step 5: Evaluation and Fine-Tuning
After training, it’s essential to evaluate the model’s performance on a validation set. This helps to ensure that the model generalizes well to unseen data. Common evaluation metrics for image captioning include BLEU, METEOR, and CIDEr scores.
Based on the evaluation results, you may need to fine-tune the model. This could involve adjusting the learning rate, adding regularization, or using more advanced techniques like learning rate scheduling or gradient clipping.
Step 6: Deployment
Once satisfied with the model's performance, the final step is deployment. The deployment process depends on the target environment—whether it's a web application, mobile app, or standalone software.
6.1 Model Export
Export the trained model in a format suitable for deployment, such as ONNX or TorchScript for PyTorch models.
torch.jit.save(torch.jit.script(model), "multimodal_model.pt")
6.2 Integration with an Application
In a typical deployment scenario, the model would be integrated into an application that provides an interface for users to upload images and enter text prompts. The model then processes this input and generates a caption.
Here’s a basic example using Flask for a web-based deployment:
from flask import Flask, request, jsonify
import torch
app = Flask(__name__)
# Load the model
model = torch.jit.load('multimodal_model.pt')
model.eval()
@app.route('/generate_caption', methods=['POST'])
def generate_caption():
image = preprocess_image(request.files['image'].read())
text = preprocess_caption(request.form['text'])
with torch.no_grad():
caption = model(text, image.unsqueeze(0))
return jsonify({'caption': tokenizer.decode(caption.argmax(dim=1).tolist())})
if __name__ == '__main__':
app.run(debug=True)
Conclusion
Building a text and image multimodal LLM involves several key steps, from data preparation and model architecture design to training, evaluation, and deployment. While the implementation can be complex, understanding each step's role in the process helps to demystify the process and makes it more approachable.
This end-to-end example should serve as a starting point for anyone looking to implement their own multimodal LLMs. With the increasing availability of multimodal datasets and pre-trained models, the barrier to entry is lower than ever, making now a great time to dive into this exciting area of AI.
This blog provides a practical, step-by-step guide to implementing a text and image multimodal LLM, offering developers the tools and knowledge needed to create powerful, integrated AI models.