Designing a Scalable Real-time Chat System: Architecture, Challenges, and Best Practices
Discover how to design a scalable real-time chat system capable of supporting millions of users. This comprehensive guide covers key architectural components, scaling strategies, and best practices for building robust chat applications.
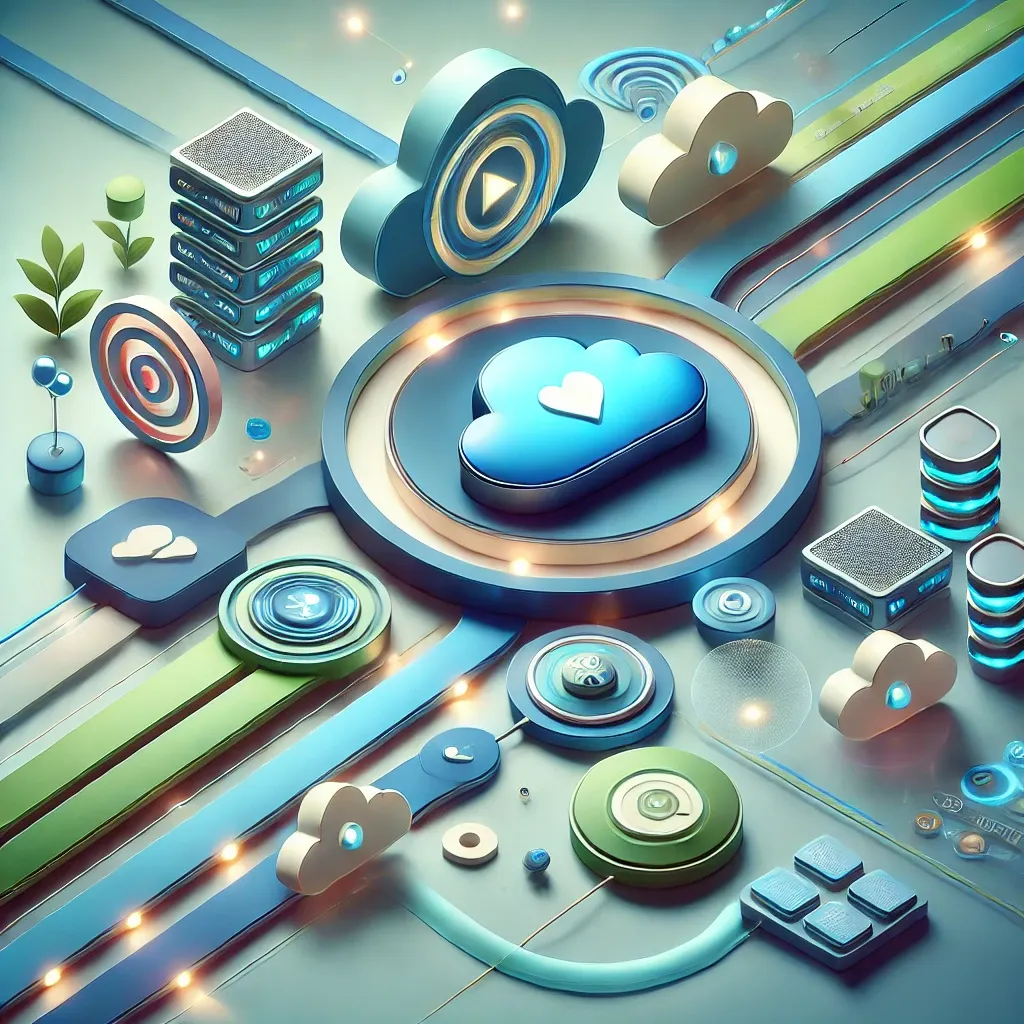
Introduction
In today's interconnected world, real-time chat systems have become an integral part of our daily lives, powering communication in applications ranging from social media platforms to customer support tools. As software developers, understanding how to design and implement a scalable chat system is a valuable skill that touches on many aspects of system design. In this blog post, we'll dive deep into the architecture, challenges, and best practices for creating a robust, real-time chat system that can handle millions of users.
Understanding the Requirements
Before we delve into the architecture, let's outline the key requirements for our chat system:
- Real-time messaging: Messages should be delivered instantly.
- Scalability: The system should support millions of concurrent users.
- Reliability: Messages should never be lost, and the system should be highly available.
- Consistency: Users should see messages in the correct order.
- Multi-device support: Users should be able to access the chat from multiple devices.
- Group chat functionality: The system should support both one-on-one and group chats.
- Message persistence: Chat history should be stored and retrievable.
High-Level Architecture
To meet these requirements, we'll design a distributed system with the following components:
- Client applications (Web, Mobile, Desktop)
- Load Balancers
- Chat Service
- Presence Service
- Notification Service
- Message Queue
- Database Cluster
- Caching Layer
Let's explore each component in detail:
Client Applications:
The client applications will be responsible for the user interface and initial message handling. They'll communicate with the backend services using WebSockets for real-time bi-directional communication. WebSockets are preferred over traditional HTTP polling as they provide a persistent connection, reducing latency and server load.
Load Balancers:
Load balancers will distribute incoming traffic across multiple server instances to ensure even distribution of load and high availability. We'll use Layer 7 (application layer) load balancing to route WebSocket connections and HTTP requests appropriately.
Chat Service:
The chat service is the core component of our system. It will handle:
- Message routing
- User authentication and authorization
- WebSocket connection management
- Message persistence
To ensure scalability, we'll design the chat service as a stateless microservice. Each instance of the chat service will be capable of handling any user's request, allowing us to scale horizontally by adding more instances as needed.
Presence Service:
The presence service will track user online/offline status and manage user sessions. It will:
- Update user status in real-time
- Manage user-to-device mappings
- Provide user status information to other services
Notification Service:
For users who are offline or have the app running in the background, we'll use a notification service to deliver push notifications. This service will integrate with platform-specific push notification services (e.g., Firebase Cloud Messaging, Apple Push Notification Service).
Message Queue:
A distributed message queue (e.g., Apache Kafka, RabbitMQ) will be used to decouple various components of our system. It will help in:
- Buffering messages during traffic spikes
- Ensuring reliable message delivery
- Enabling asynchronous processing
Database Cluster:
For message persistence and user data storage, we'll use a combination of databases:
a) NoSQL database (e.g., Cassandra, MongoDB):
- Store chat messages and chat room information
- Provide high write throughput and horizontal scalability
b) Relational database (e.g., PostgreSQL):
- Store user profiles and authentication data
- Handle complex queries and transactions
Caching Layer:
To reduce database load and improve response times, we'll implement a distributed caching layer (e.g., Redis, Memcached). This will cache:
- Recent messages
- User session data
- Frequently accessed user information
Detailed Component Interactions:
Now that we've outlined the major components, let's walk through how they interact in various scenarios:
a) Sending a message:
- The client sends a message via WebSocket to the chat service.
- The chat service authenticates the user and validates the message.
- The message is published to the message queue.
- The chat service persists the message in the NoSQL database.
- The message is sent to online recipients via WebSocket.
- For offline recipients, a push notification is sent via the notification service.
b) Receiving a message:
- Online users receive messages directly via their WebSocket connection.
- Offline users receive push notifications.
- When a user comes online, recent messages are fetched from the cache or database.
c) User comes online:
- The client establishes a WebSocket connection with the chat service.
- The chat service notifies the presence service of the user's online status.
- The presence service updates the user's status in the cache and database.
- The chat service fetches recent messages for the user from the cache or database.
Scaling Considerations:
To handle millions of concurrent users, we need to consider several scaling strategies:
a) Horizontal Scaling:
- Deploy multiple instances of the chat service, presence service, and notification service behind load balancers.
- Shard the database to distribute data across multiple nodes.
b) Message Partitioning:
- Partition messages by chat room or user ID to distribute the load across multiple database nodes.
c) Caching Strategy:
- Implement multi-level caching (application-level and distributed cache) to reduce database load.
- Use cache aside pattern for reading data and write-through cache for updates.
d) Connection Handling:
- Optimize WebSocket connections using connection pooling.
- Implement a connection draining mechanism during deployments to ensure smooth transitions.
e) Database Optimization:
- Use database read replicas to handle read-heavy operations.
- Implement database indexing strategies to optimize query performance.
Handling Edge Cases and Challenges:
Several challenges need to be addressed to ensure a robust chat system:
a) Message Ordering:
- Use logical clocks (e.g., Lamport timestamps) to maintain message order across distributed systems.
b) Consistency:
- Implement eventual consistency for message delivery.
- Use techniques like conflict-free replicated data types (CRDTs) for handling concurrent edits in group chats.
c) Offline Message Delivery:
- Store undelivered messages in a separate queue for offline users.
- Implement a message delivery retry mechanism with exponential backoff.
d) Large Group Chats:
- Implement fan-out on read for very large group chats to reduce write amplification.
- Use pagination and lazy loading for fetching chat history.
e) Media Sharing:
- Use a content delivery network (CDN) for efficient delivery of shared media files.
- Implement progressive loading for large media files.
f) Security:
- Implement end-to-end encryption for message content.
- Use secure WebSocket connections (WSS) for client-server communication.
- Implement rate limiting to prevent abuse and DDoS attacks.
Monitoring and Observability:
To maintain a healthy system, implement comprehensive monitoring:
a) Real-time Metrics:
- Message delivery rates
- WebSocket connection counts
- Service response times
b) Logging:
- Implement distributed tracing to track message flow across services.
- Use log aggregation tools for centralized log management.
c) Alerting:
- Set up alerts for anomalies in message delivery rates, error rates, and system resource utilization.
Testing Strategies:
Ensure system reliability through thorough testing:
a) Unit Testing:
- Test individual components and functions in isolation.
b) Integration Testing:
- Test interactions between different services.
c) Load Testing:
- Simulate high concurrency to verify system performance under load.
d) Chaos Engineering:
- Randomly inject failures to ensure system resilience.
Conclusion
Designing a scalable real-time chat system involves careful consideration of various components and their interactions. By leveraging a microservices architecture, distributed databases, caching, and message queues, we can create a robust system capable of handling millions of users.
Key takeaways from this design include:
- Use WebSockets for real-time communication.
- Implement a distributed architecture for scalability.
- Leverage caching to reduce database load.
- Use message queues for reliable and asynchronous processing.
- Consider eventual consistency and handle edge cases.
- Implement comprehensive monitoring and testing strategies.
Remember that system design is an iterative process. As your chat application grows, you may need to revisit and optimize various components of the architecture. Stay informed about new technologies and best practices, and be prepared to evolve your system to meet changing requirements and scale.
By following these principles and continuously refining your approach, you'll be well-equipped to build and maintain a scalable real-time chat system that can serve millions of users effectively.