The Art of Clean Code: Best Practices for Writing Maintainable Software
Discover the art of clean code: Learn key principles like readability, simplicity, and SOLID. Get practical tips for writing maintainable functions, handling errors, and organizing your codebase. Elevate your coding practices with real-world examples and tool recommendations.
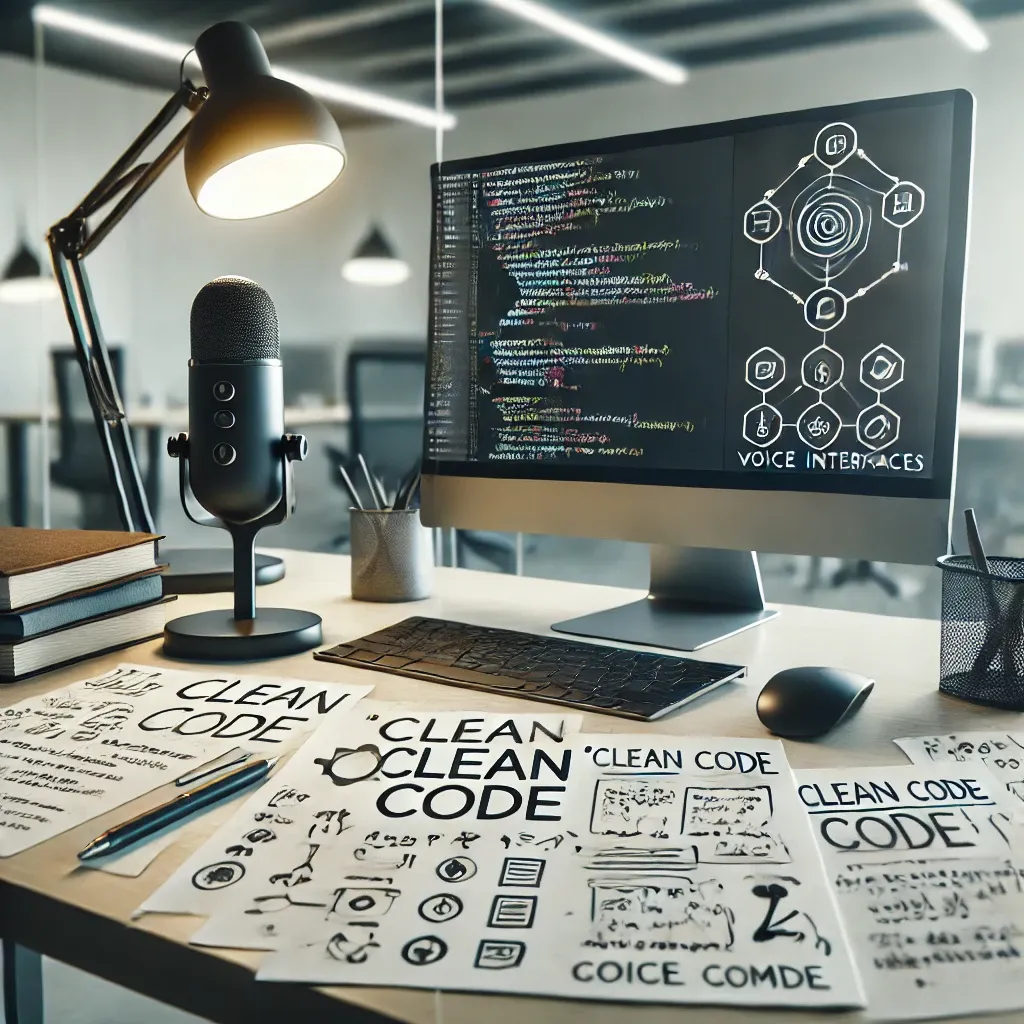
Introduction
In the world of software development, writing code that works is just the beginning. As projects grow in size and complexity, the ability to write clean, maintainable code becomes increasingly crucial. Clean code isn't just about aesthetics; it's about creating software that's easy to understand, modify, and debug. In this article, we'll explore the principles of clean code and provide practical tips to help you improve your coding practices.
What is Clean Code?
Clean code is code that is easy to read, understand, and maintain. It's code that clearly expresses its intent, follows consistent patterns, and minimizes complexity. Robert C. Martin, author of "Clean Code: A Handbook of Agile Software Craftsmanship," describes it as code that looks like it was written by someone who cares.
Key Principles of Clean Code
Readability
Readability is perhaps the most fundamental aspect of clean code. Your code should be easy to read and understand, not just for you but for other developers who might work on the project in the future.
Tips for improving readability:
- Use meaningful and pronounceable variable names
- Write descriptive function names
- Keep functions and classes small and focused
- Use consistent formatting and indentation
- Add comments sparingly, only when necessary to explain complex logic
Simplicity
Clean code strives for simplicity. It does one thing well, rather than trying to solve multiple problems at once.
Tips for maintaining simplicity:
- Follow the Single Responsibility Principle (SRP)
- Avoid premature optimization
- Refactor complex code into smaller, more manageable pieces
- Use built-in language features and standard libraries when possible
DRY (Don't Repeat Yourself)
The DRY principle states that every piece of knowledge or logic should have a single, unambiguous representation within a system.
Tips for staying DRY:
- Extract repeated code into functions or methods
- Use inheritance and composition to share common functionality
- Implement design patterns to solve recurring problems
SOLID Principles
The SOLID principles are a set of five design principles that help create more maintainable and extendable software:
- Single Responsibility Principle (SRP)
- Open-Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Adhering to these principles can significantly improve the quality and maintainability of your code.
Consistent Naming Conventions
Consistency in naming conventions makes code more predictable and easier to read.
Tips for consistent naming:
- Use camelCase for variables and functions in languages like JavaScript
- Use PascalCase for class names
- Use UPPERCASE for constants
- Choose descriptive names that reveal intent
Proper Code Organization
Well-organized code is easier to navigate and understand.
Tips for better organization:
- Group related functions and classes together
- Use modules or namespaces to avoid polluting the global scope
- Keep files focused on a single concept or component
- Follow a consistent directory structure for your project
Error Handling
Proper error handling is crucial for creating robust and maintainable software.
Tips for effective error handling:
- Use try-catch blocks to handle exceptions
- Provide meaningful error messages
- Avoid using exceptions for flow control
- Log errors for debugging purposes
Writing Clean Functions
Functions are the building blocks of your code. Clean functions are essential for maintaining overall code quality.
Tips for writing clean functions:
- Keep functions small and focused on a single task
- Limit the number of parameters (ideally 3 or fewer)
- Avoid side effects when possible
- Use meaningful names that describe what the function does
Comments and Documentation
While clean code should be largely self-explanatory, comments and documentation still play a crucial role.
Tips for effective commenting:
- Use comments to explain why, not what (the code should be self-explanatory)
- Keep comments up-to-date as code changes
- Use documentation generators for APIs and libraries
- Write meaningful commit messages
Testing
Clean code is testable code. Writing tests not only helps ensure your code works as expected but also serves as documentation and aids in refactoring.
Tips for effective testing:
- Write unit tests for individual functions and methods
- Use test-driven development (TDD) when appropriate
- Aim for high test coverage, especially for critical parts of your codebase
- Keep tests clean and maintainable, just like production code
Practical Examples of Clean Code
Let's look at some before-and-after examples to illustrate clean code principles:
Example 1: Improving Function Naming and Responsibility
Before:
def process(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
return result
After:
def double_even_numbers(numbers):
return [num * 2 for num in numbers if num % 2 == 0]
In this example, we've improved the function name to clearly describe its purpose. We've also simplified the logic using a list comprehension, making the code more concise and readable.
Example 2: Applying the Single Responsibility Principle
Before:
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def save(self):
# Code to save user to database
pass
def send_email(self, subject, body):
# Code to send email
pass
After:
class User:
def __init__(self, name, email):
self.name = name
self.email = email
class UserRepository:
def save(self, user):
# Code to save user to database
pass
class EmailService:
def send_email(self, to_email, subject, body):
# Code to send email
pass
In this refactored version, we've separated the responsibilities of the User class into distinct classes, adhering to the Single Responsibility Principle.
Example 3: Improving Error Handling
Before:
def divide(a, b):
return a / b
result = divide(10, 0) # This will raise an uncaught ZeroDivisionError
After:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
try:
result = divide(10, 0)
except ValueError as e:
print(f"Error: {e}")
# Handle the error appropriately
In this improved version, we've added proper error handling to make the code more robust and informative.
Tools for Maintaining Clean Code
Several tools can help you maintain clean code:
- Linters: Tools like ESLint (JavaScript), Pylint (Python), or RuboCop (Ruby) can automatically check your code for style and potential errors.
- Formatters: Tools like Prettier (JavaScript) or Black (Python) can automatically format your code to a consistent style.
- Code Analysis Tools: SonarQube, CodeClimate, and similar tools can provide deeper insights into code quality and potential issues.
- Version Control: Using Git with meaningful commit messages and proper branching strategies helps maintain a clean and organized codebase.
- Continuous Integration (CI): Implementing CI pipelines can automate testing and ensure code quality standards are met before merging changes.
The Benefits of Clean Code
Investing time in writing clean code pays off in numerous ways:
- Improved Maintainability: Clean code is easier to understand and modify, reducing the time and effort required for maintenance.
- Enhanced Collaboration: When code is clean and well-organized, it's easier for team members to work together and contribute to the project.
- Reduced Bugs: Clean code is often less prone to bugs and easier to debug when issues do arise.
- Faster Development: While writing clean code might take more time initially, it speeds up development in the long run by making the codebase easier to work with.
- Better Scalability: Clean code provides a solid foundation for growing and scaling your application.
- Improved Performance: Clean code often leads to more efficient code, as it's easier to identify and optimize bottlenecks.
Conclusion
Writing clean code is a skill that takes time and practice to develop. It's not about perfection, but about consistently striving to improve the quality of your code. By following the principles and practices outlined in this article, you can significantly enhance the readability, maintainability, and overall quality of your codebase.
Remember, clean code is a continuous process. As you write and review code, always ask yourself: "Can this be clearer? Can it be simpler? How would another developer interpret this?" By constantly questioning and refining your code, you'll not only improve your own skills but also contribute to creating better, more maintainable software.
Clean code isn't just about following a set of rules; it's about cultivating a mindset of craftsmanship in software development. It's about taking pride in your work and considering the impact of your code on your team and your future self. As you continue your journey in software development, let the principles of clean code guide you towards creating elegant, efficient, and enduring solutions.